binary tree python geeksforgeeks
Create a binary search tree in which each node stores the following information of a person. 3 Traverse both paths till the values in arrays are the same.
Introduction To Tree Data Structure Geeksforgeeks
Inorder Successor of a node in Binary Tree.
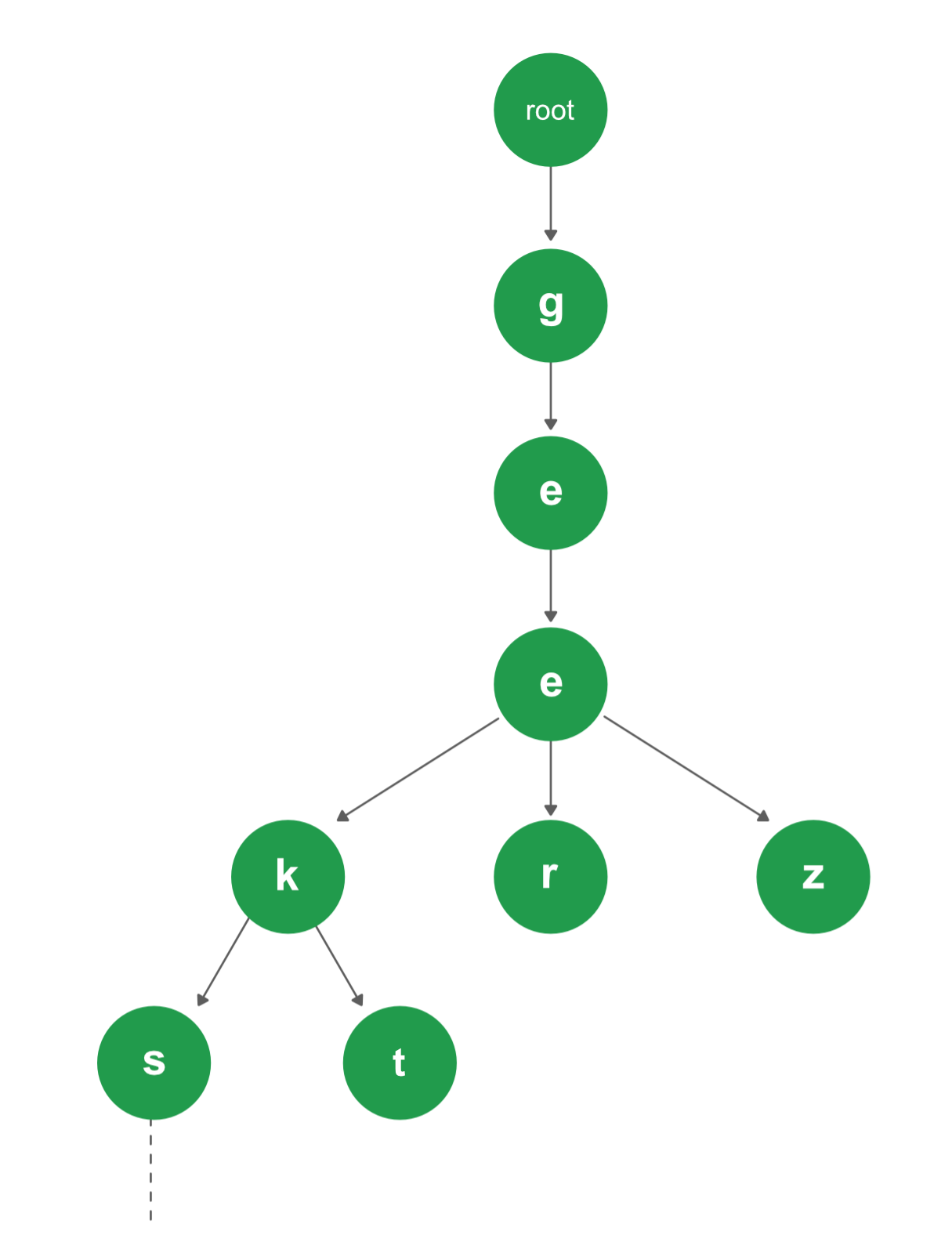
. Given a binary tree find its height. See this for step wise step execution of the algorithm. Replace each node in binary tree with the sum of its inorder predecessor and successor.
1 2 3 Output. Binary tree python geeksforgeeks. Your task is to complete the function height which takes root node of the tree as input parameter and returns an integer denoting the height of the treeIf the tree is empty return 0.
Tree was given in the form. Otherwise output is 0. If the tree is empty return 0.
2 Initialize current node as root 3 Push the current node to S and set current current-left until current is NULL 4 If current is NULL and stack is not empty then a Pop the top item from stack. For Binary search tree while traversing the tree from top to bottom the first node which lies in between the two numbers n1 and n2 is the LCA of the nodes ie. 2 1 3 Output.
2 1 3 Output. Given level order traversal of a Binary Tree check if the Tree is a Min-Heap. The return type is cpp.
Root node of the binary tree. Expression Tree Easy Accuracy. How the search for an element in a binary search tree.
The value of the root node index would always be -1. Check if given Preorder Inorder and Postorder traversals are of same tree. Tree generates a random binary tree and returns its root node.
Please try your approach on. You dont need to read input or print anything. 1 2 3 Output.
It is the height of the tree and its value can be between the range 0-9 inclusive is_perfect. How the search for an element in a binary search tree. 1 2 3 4 Explanation.
Your task is to complete the function binaryTreeToBST which takes the root of the Binary tree as input and returns the root of the BST. The sums of these three paths are 16 4 and 17 respectively. The first node n with the lowest depth which lies in between n1 and n2 n1.
Your task is to complete the function Ancestors that finds all the ancestors of the key in the given binary tree. 1 2 3 Output. Complete the function cloneTree which takes root of the given tree as input parameter and returns the root of the cloned tree.
If the tree is empty then value of root is NULL. Given a binary tree find its height. Following is the implementation of the above algorithm.
No need to read input or print anything. You dont need to read input or print anything. So just recursively traverse the BST in if nodes value is greater than both n1 and n2 then our LCA lies.
Name age NID and height. Return the common element just before the mismatch. Binary tree python geeksforgeeks.
The left and right subtree each must also be a binary search tree. Create a binary search tree in which each node stores the following information of a person. Find all possible binary trees with given Inorder Traversal.
Name age NID and height. Since each element in a binary tree can have only 2 children we typically name them the left and right child. Given a binary tree the task is to flip the binary tree towards the right direction that is clockwise.
2 Given a full binary expression tree consisting of basic binary operators and some integers Your task is to evaluate the expression tree. You dont need to read input or print anything. The output is 1 if the tree is cloned successfully.
The array indexes are values in tree nodes and array values give the parent node of that particular index or node. Construct Binary Tree from Parent Array. 2 Find a path from the root to n2 and store it in another vector or array.
Binary Tree Representation in C. Given a Binary Tree find the maximum sum path from a leaf to root. 1 2 3 4 Output.
1 Find a path from the root to n1 and store it in a vector or array. Given the root of a binary tree in which all nodes has values 0 or 1 the task is to find and print all levels for which another level exists such that the decimal representation of each is sameIf no such level exists return an empty list. Level Order Tree Traversal.
For example in the following tree there are three leaf to root paths 8--2-10 -4--2-10 and 7-10. X Y Z indicates Y and Z are the left and right sub stress respectively of node X. 1 Create an empty stack S.
Populate Inorder Successor for all nodes. Check if two nodes are cousins in a Binary Tree. What is an ADT Write an algorithm to insert an element into BST.
In the flip operation the leftmost node becomes the root of the flipped tree and its parent becomes its right child and the right sibling becomes its left child and the same. Given a binary tree and a key insert the key into the binary tree at the first position available in level order. Check if all leaves are at same level.
The right subtree of a node contains only nodes with keys greater than the nodes key. Note that Y and Z may be NULL or further nested. The converted BST will be 3 2 4 1.
A tree is represented by a pointer to the topmost node in tree. Build a random binary tree. Flip Binary Tree.
See the below examples to see the transformation. Given an array of size N that can be used to represents a tree. H is the height of the tree and this space is used implicitly for the recursion stack.
Find n-th node of inorder traversal. Binary Search Tree is a node-based binary tree data structure which has the following properties. What is an ADT Write an algorithm to insert an element into BST.
Your task is to complete the function height which takes root node of the tree as input parameter and returns an integer denoting the height of the tree. Given a level K you have to find out the sum of data of all the nodes at level K in a binary tree. The tree will be created based on the height of each person.
Clone the given tree. If set True a perfect binary is created. Below is an algorithm for traversing binary tree using stack.
Consider the following nested representation of binary trees. Check if removing an edge can divide a Binary Tree in two halves. 1 0 1.
Which of the following represents a valid binary tree. A tree whose elements have at most 2 children is called a binary tree. The tree was cloned successfully.
Below are the various methods used to create and perform various operations on a. In order to create a binary tree we first import the dstructure module create a BTree class object to initialize an empty binary tree and use the insert method to insert nodes into the tree. 0 5 7 6 4 1 3 9.
The left subtree of a node contains only nodes with keys lesser than the nodes key.
Python Requests Get Post Url Computer Science Interview Questions Sql Tutorial
Selenium Python Tricks Geeksforgeeks Content Writing Writing Article Writing
Python Document Field Detection Using Template Matching Geeksforgeeks Algorithm Interview Questions Computer Science
Diagonal Traversal Of Binary Tree Geeksforgeeks
Tree Question Solution From Geeksforgeeks Problem Solving Solving Data Visualization
Binary Trees Introduction Geeksforgeeks Youtube
Binary Indexed Tree Or Fenwick Tree Geeksforgeeks Computer Science Time Complexity Fenwick
Tutorial On Binary Search Tree Geeksforgeeks
Flood Fill Algorithm How To Implement Fill In Paint Geeksforgeeks Flood Fill Logical Thinking Algorithm
Red Black Tree Red Black Tree Binary Tree Black Tree
Construct Tree From Given Inorder And Preorder Traversals Geeksforgeeks Pre Order Algorithm Construction
Tutorial On Binary Tree Geeksforgeeks
Check Whether A Binary Tree Is A Full Binary Tree Or Not Geeksforgeeks
Binary Tree Using Dstructure Library In Python Geeksforgeeks
Difference Between General Tree And Binary Tree Geeksforgeeks
Top Three Elements In Binary Tree Geeksforgeeks Youtube